Device dashboard on NUCLEO-F746ZG - CMSIS-RTOS v2 + lwIP, using Keil MDK
Overview
This tutorial shows how to implement a Web device dashboard using Mongoose Library over CMSIS-RTOS v2 + lwIP on an STM32 Nucleo-F746ZG development board, using the ARM Keil MDK development environment.
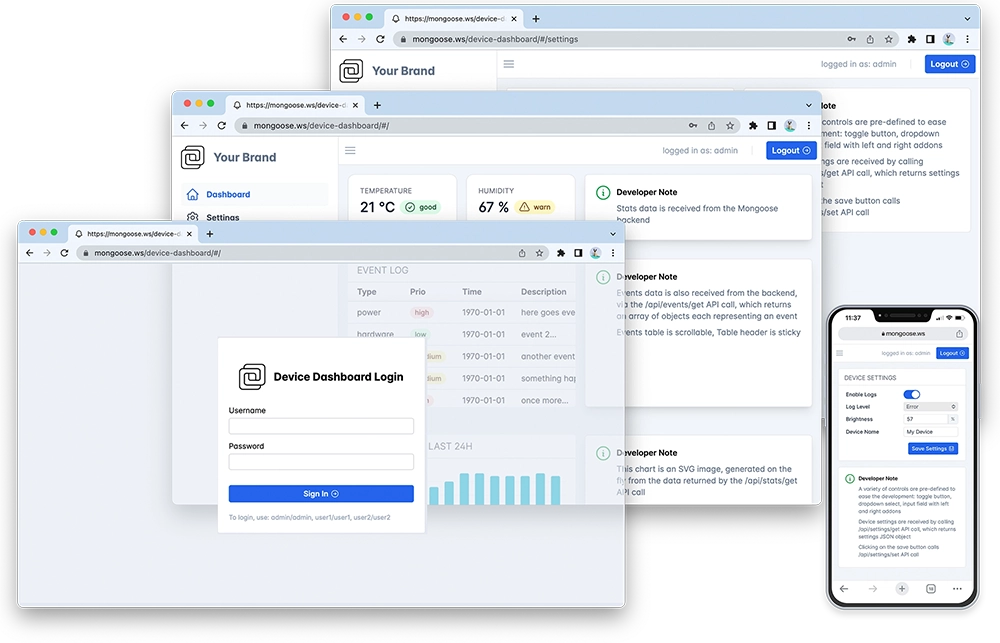
Features of this implementation include:
- Uses Keil RTX5, lwIP, ARM CMSIS Core, ARM CMSIS Driver, and device headers through Software Packs
- The Web dashboard provides:
- User Authentication: login protection with multiple permission levels
- The web UI is optimized for size and for TLS usage
- Logged users can view/change device settings
- The web UI is fully embedded into the firmware binary, and does not need a filesystem to serve it, making it resilient
This example is a hardware adaptation of the Device Dashboard that can run on Mac/Linux/Windows. Mongoose Library, being cross-platform, allows to develop and run the same code on different platforms. That means: all functionality related to networking can be developed and debugged on a workstation, and then run as-is on an embedded device - and this example is a demonstration of that.
Take your time to navigate and study the Device Dashboard tutorial. Here, we concentrate on the features specific to this embedded platform in the Keil MDK environment.
The Keil RTX5 implementation of this project is at tutorials/stm32/nucleo-f746zg-keil-rtx5-lwip
. Though we specifically refer to this implementation, most references in this tutorial are valid for any supported RTOS. However, to use an RTOS other than Keil RTX5, please check this section.
This example is a plain Keil MDK-based project with the following files of interest:
- main.c - provides the
main()
entry point with hardware init, LED blinking and network init. - syscalls.c - provides a low level function to redirect debug output to a UART.
mongoose.c
,mongoose.h
- Mongoose Librarynet.c
,net.h
- part of the device dashboard example, contains the Web functionalitypacked_fs.c
- part of the device dashboard example, embeds the Web UI used by the dashboard
All these files have been grouped in Source Group 1
in the Project Explorer. Keil MDK can integrate with STM32CubeMX, and we use it. The device configuration file, used as the recipe to generate the code for device initialization, is handled by the IDE, we access it through the Run-Time Environment manager. For all auto-generated files, we've used those places designated for USER_CODE, so all files can be re-generated by STM32CubeMX for newer versions of the firmware packs.
References in this tutorial are for a Nucleo-F746ZG board
Below is a general process outline:
- The board IP addressing will be provided by a DHCP server. If you want to set a static configuration, see below
- Build the example (see below) and run it on a development board
- The firmware initializes the RTOS; see RTOS integration below
- The firmware initializes the network; see lwIP integration below
- After initialization, the application starts Mongoose's event loop and blinks a blue LED
- Once the blue LED starts blinking, the example is ready
- Open your web browser and navigate to the board IP address, you should see a nice device dashboard
Build and run
Follow the Build Tools tutorial to setup your development environment.
In your project directory, clone the Mongoose Library repository using git
Start μVision and open the project; if you need a quick start on the ARM Keil MDK and μVision, follow this step by step tutorial.
To be able to build this project, you need to have the proper Software Packs to support it. If you don't have already installed the
Keil::MDK-Middleware
pack (and its dependencies) and thelwIP::lwIP
pack, you'll see a requester asking you permission to do so. The Pack Installer will open and install the packs.In order to build this project, click the
Build
icon. To flash this firmware to your board, plug it in a USB port and click on theLoad
icon. When finished, you have to reset your board pressing its reset button. You should soon see the blue LED start blinking. As long as there is only one board plugged in, μVision will find it; though we need to know the serial port device to be able to get the log information. You'll need to dig for it in your computer.When the firmware starts, the board should signal its state by blinking the blue LED. We now need to know the IP address of the board to connect to it. If we used DHCP, as it is the default, we can check our DHCP server logs or see the device logs. Let's do this.
To connect to the board, in this example we'll be using PuTTY; we configure it for 115200bps.
0 2 main.c:73:app_main Waiting for IP... 1202 2 main.c:76:app_main READY, IP: 192.168.69.241 1212 2 main.c:30:server Initialising application... 121d 3 mongoose.c:3356:mg_listen 1 0x0 http://0.0.0.0 1228 2 main.c:34:server Starting event loop
Now start a browser on
http://IP_ADDRESS
, whereIP_ADDRESS
is the board's IP address printed on the serial console. You should see a login screen as in the image aboveFrom here on, if you want to try the dashboard features please go to the device dashboard tutorial and follow some of the steps depicted there.
Compilation options
MG_ARCH = MG_ARCH_CMSIS_RTOS2
- configures Mongoose to work with a supported RTOS using CMSIS-RTOS API v2MG_ENABLE_LWIP = 1
- enables the lwIP stackMG_ENABLE_CUSTOM_RANDOM = 1
- lets the firmware code overridemg_random()
to use the device hardware RNGMG_ENABLE_PACKED_FS = 1
- enables the embedded filesystem support
These are grouped in the file mongoose_config.h. This file has been written using scripting extensions, so you can just select the Configuration Wizard tab at the bottom of the editor to see the file contents in a friendly way.
main.c overview
This example can be divided in the following blocks:
- CMSIS-RTOS v2 integration
- lwIP integration
- Initialize the microcontroller for this particular board and take advantage of the true RNG in the microcontroller
- Start the scheduler to run the desired tasks
- Initialize the networking stack
- Initialize Mongoose
- Run Mongoose
RTOS integration
Mongoose supports a number of well-known architectures, among them CMSIS-RTOS v2. To tell Mongoose in which architecture it is running, we need to define the macro MG_ARCH
as we've seen above; we do this in mongoose_config.h
.
- We follow our basic RTOS integration guidelines, as these still apply. See OS specifics below
lwIP integration
Mongoose supports a number of well-known TCP/IP stacks, among them lwIP. To tell Mongoose which stack to use, we need to define a macro as we've seen above; we do this in mongoose_config.h
.
- Follow the basic lwIP integration guidelines tutorial.
Custom mg_random()
Some network operations require the generation of random numbers, from simple port numbers that should be different on every reset to complex TLS operations. Mongoose supports a number of well-known architectures, but since here we are working at the bare-metal level, we need to provide our own custom function or default to the standard pseudo-random number generator. The necessary actions are:
- Define
MG_ENABLE_CUSTOM_RANDOM=1
which is done inmongoose_config.h
- Provide a custom
void mg_random(void *buf, size_t len)
function:
In this example, this function uses the microcontroller's built-in RNG through the STM32Cube HAL provided in the Keil packs
MCU and board initialization
Microcontroller support is provided by Keil firmware packs, which are based on STM32Cube firmware packages. The microcontroller, its clock, and all peripherals we use are initialized by HAL functions according to the configuration, code like this is generated by STM32CubeMX. In fact, to reproduce all the configuration steps, follow the step by step tutorial up to and including step 5. In particular, step 1 describes the additions we need to do to a Cube-generated main file to call the generated initialization functions, this mx_init()
function we call at the start of our main() function.
Since this is a CMSIS-RTOS v2 project, task stack allocation will be done through it; dynamic memory allocation still will be done through system calls so we need a system heap.
We've left the initial stack allocation at a small value (1KB), and setup enough heap room at project creation; that can be seen/modified by opening the assembly startup file (Device/startup_<devicefamilyname>.s
) in μVision and then use the Configuration Wizard. This setting affects the linker process.
Create tasks and start scheduler
We create the initial tasks and call the function that starts the scheduler. Then, we have an app_main task, where we initialize the network and create the server task that runs Mongoose, and a blinker task to blink an LED
TCP/IP initialization
Our app_main task initializes the network, creates a task to poll for link changes and to serve the ethernetif, then polls the IP address until it has a valid value (the DHCP server assigns us an address). Then it will create the server task to run Mongoose, and terminate.
Note we provide ample stack space for the Mongoose task. It doesn't actually need to be that big for such a simple example, but more complex interfaces will need plenty of room.
Mongoose initialization
In the server task we initialize Mongoose; this is no different from what we always do in any example; we are a task in an RTOS and we can run an infinite loop.
Run Mongoose
Then we run Mongoose. This is no different from what we always do in any example, though note that it should be run after network initialization. The logic is standard: initialize the event manager (as we already did), start a listener, and fall into an infinite event loop:
In this case, the listener is started by web_init()
, the device dashboard initialization function. The URL is configured by the macro HTTP_URL
, which we defined as a preprocessor symbol.
Blinker task
This is a simple task that toggles the GPIO and loops, to blink the blue LED
We have covered those aspects that are specific to the STM32 implementation, for the details on the application and the UI, please see the Device Dashboard tutorial.
Differences among supported RTOSes
The FreeRTOS implementation is at tutorials/stm32/nucleo-f746zg-keil-freertos_cmsis2-lwip
. In this example only configuration files change; but we need to pay special attention to task termination:
- When using FreeRTOS, do not loop but exit on task termination:
Make sure you setup a minimum stack size of 256 words, otherwise lwIP may not even start
- in
RTOS/FreeRTOSConfig.h
setMinimal stack size [words] 256
At the RTOS integration section we also linked you to basic setup guidelines for supported RTOSes, make sure you check them